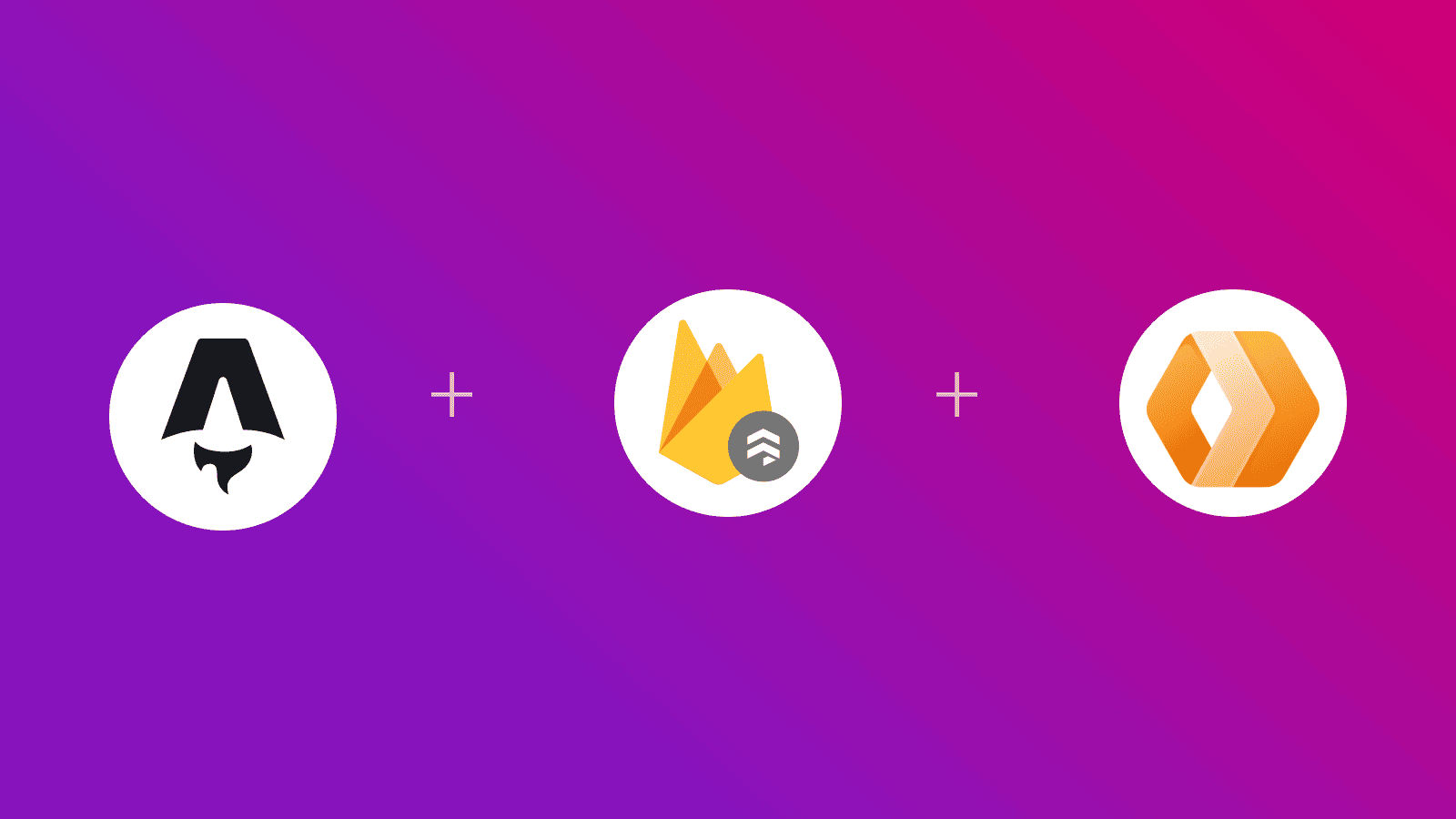
In this guide, you will learn how to interact with Cloud Firestore instance from an Astro application running on Cloudflare Workers. You will go through the process of setting up a new Astro project, enabling server-side rendering using Cloudflare adapter, installing the necessary packages, writing code to interact with Cloud Firestore, and deploy the application to Cloudflare Workers.
Prerequisites
- Node.js 20 or later
- A Firebase account
Table Of Contents
- Generate a Firebase Service Account JSON
- Create a new Astro application
- Integrate Cloudflare adapter in your Astro project
- Access the Environment Variables
- Initialize Firebase Connection
- Query Cloud Firestore from Astro application
- Deploy to Cloudflare Workers
Generate a Firebase Service Account JSON
- Go to the Firebase Console and browse your project (create one if you do not have one already).
- In the Firebase Console, navigate to Project Settings by clicking the gear icon.
- Next, go to the Service accounts tab.
- Finally, click on Generate new private key and download the JSON file. This file contains your Firebase service account credentials.
Create a new Astro application
Let’s get started by creating a new Astro project. Open your terminal and run the following command:
npm create astro@latest my-app
npm create astro
is the recommended way to scaffold an Astro project quickly.
When prompted, choose:
Use minimal (empty) template
when prompted on how to start the new project.Yes
when prompted to install dependencies.Yes
when prompted to initialize a git repository.
Once that’s done, you can move into the project directory and start the app:
cd my-appnpm run dev
The app should be running on localhost:4321. Next, execute the command below to install the necessary library for building the application:
npm install fireworkers
The following library is installed:
fireworkers
: A library that facilitates interaction with Cloud Firestore from Cloudflare Workers.
Integrate Cloudflare adapter in your Astro project
To deploy your Astro project to Cloudflare Workers, you need to install the corresponding adapter. Execute the command below:
npx astro add cloudflare
When prompted, choose the following:
Y
when prompted whether to install the Cloudflare dependencies.Y
when prompted whether to make changes to Astro configuration file.
You have succesfully enabled server-side rendering in Astro.
To make sure that the output is deployable to Cloudflare Workers, create a wrangler.toml
file in the root of the project with the following code:
name = "cloud-firestore-astro-workers"main = "dist/_worker.js"compatibility_date = "2025-04-01"compatibility_flags = [ "nodejs_compat" ]
[assets]directory="dist"binding="ASSETS"
[vars]FIREBASE_PROJECT_ID=""FIREBASE_PRIVATE_KEY=""FIREBASE_CLIENT_EMAIL=""FIREBASE_PRIVATE_KEY_ID=""
Post that, make sure that you have both an .env
file and a wrangler.toml
file with the variables defined so that they can be accessed during npm run dev
and when deployed on Cloudflare Workers respectively.
Further, update the astro.config.mjs
file with the following to be able to access these variables in code programmatically:
// ... Existing imports...import { defineConfig, envField } from 'astro/config'
export default defineConfig({ env: { schema: { FIREBASE_PROJECT_ID: envField.string({ context: 'server', access: 'secret', optional: false }), FIREBASE_PRIVATE_KEY: envField.string({ context: 'server', access: 'secret', optional: false }), FIREBASE_CLIENT_EMAIL: envField.string({ context: 'server', access: 'secret', optional: false }), FIREBASE_PRIVATE_KEY_ID: envField.string({ context: 'server', access: 'secret', optional: false }), } } // adapter})
Access the Environment Variables
To securely access the necessary environment variables at runtime, you should use the getSecret
function from astro:env/server
. This approach, recommended from Astro 5.6 onwards, ensures that your application remains provider agnostic and sensitive information is not hardcoded. The following variables will be retrieved using this method:
- FIREBASE_PROJECT_ID
- FIREBASE_PRIVATE_KEY
- FIREBASE_CLIENT_EMAIL
- FIREBASE_PRIVATE_KEY_ID
import { getSecret } from 'astro:env/server'
const project_id = getSecret('FIREBASE_PROJECT_ID')const private_key = getSecret('FIREBASE_PRIVATE_KEY')const client_email = getSecret('FIREBASE_CLIENT_EMAIL')const private_key_id = getSecret('FIREBASE_PRIVATE_KEY_ID')
export default function getFirebaseConfig() { return { project_id, private_key, client_email, private_key_id, }}
Initialize Firebase Connection
In your Astro application, create a file named index.ts
in the src/storage/firebase
directory to export a function that will establish a connection with Cloud Firestore. Use the code below:
import getFirebaseConfig from './config'import * as Firestore from 'fireworkers'import { getSecret } from 'astro:env/server'
const firebaseConfig = getFirebaseConfig()
export async function initializeFirebase(): Promise<Firestore.DB> { return await Firestore.init({ uid: 'user1234', project_id: firebaseConfig.project_id, private_key: firebaseConfig.private_key, client_email: firebaseConfig.client_email, private_key_id: firebaseConfig.private_key_id, })}
export { Firestore }
The code above imports the getSecret
function from astro:env/server
to access environment variables dynamically during runtime. It then imports the getFirebaseConfig
function to retrieve Firebase configuration details. The initializeFirebase
function is defined to initialize a Firestore database connection using the configuration details obtained from getFirebaseConfig
. Finally, the Firestore
module is exported for use in other parts of the application.
Query Cloud Firestore from Astro application
Now, you are ready to execute commands on Firestore by using the firestore
instance. In your Astro page, write the code below to interact with Cloud Firestore:
---import { initializeFirebase, Firestore } from '../../storage/index'
const firestore = await initializeFirebase()
// To set a document in `storage` collection, await Firestore.set(firestore, 'storage', key, { value }, { merge: true })// To remove a document in the `storage` collection, await Firestore.remove(firestore, 'storage', key)
// Get the document (of key `productsCount`) and use it's value property (from the `storage` collection)let count = await Firestore.get(firestore, 'storage', 'productsCount').then((doc) => doc.fields.value).catch(() => null)if (!count) count = 0---
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"></head><body> {count}</body></html>
This code imports the initializeFirebase
function and the entire Firestore
exported and retrieves the value associated with the key productsCount
from Cloud Firestore using the .get()
method.
Deploy to Cloudflare Workers
To make your application deployable to Cloudflare Workers, create a file named .assetsignore
in the public
directory with the following content:
_routes.json_worker.js
Next, you will need to use the Wrangler CLI to deploy your application to Cloudflare Workers. Run the following command to deploy:
npm run build && npx wrangler@latest deploy
References
Conclusion
In this blog post, you learned how to query Cloud Firestore from an Astro application running on Cloudflare Workers. You went through the steps of setting up the Cloud Firestore client, establishing a connection, and executing commands to retrieve data from Firestore within the Astro application.
If you would like to explore specific sections in more detail, expand on certain concepts, or cover additional related topics, please let me know, and I’ll be happy to assist!