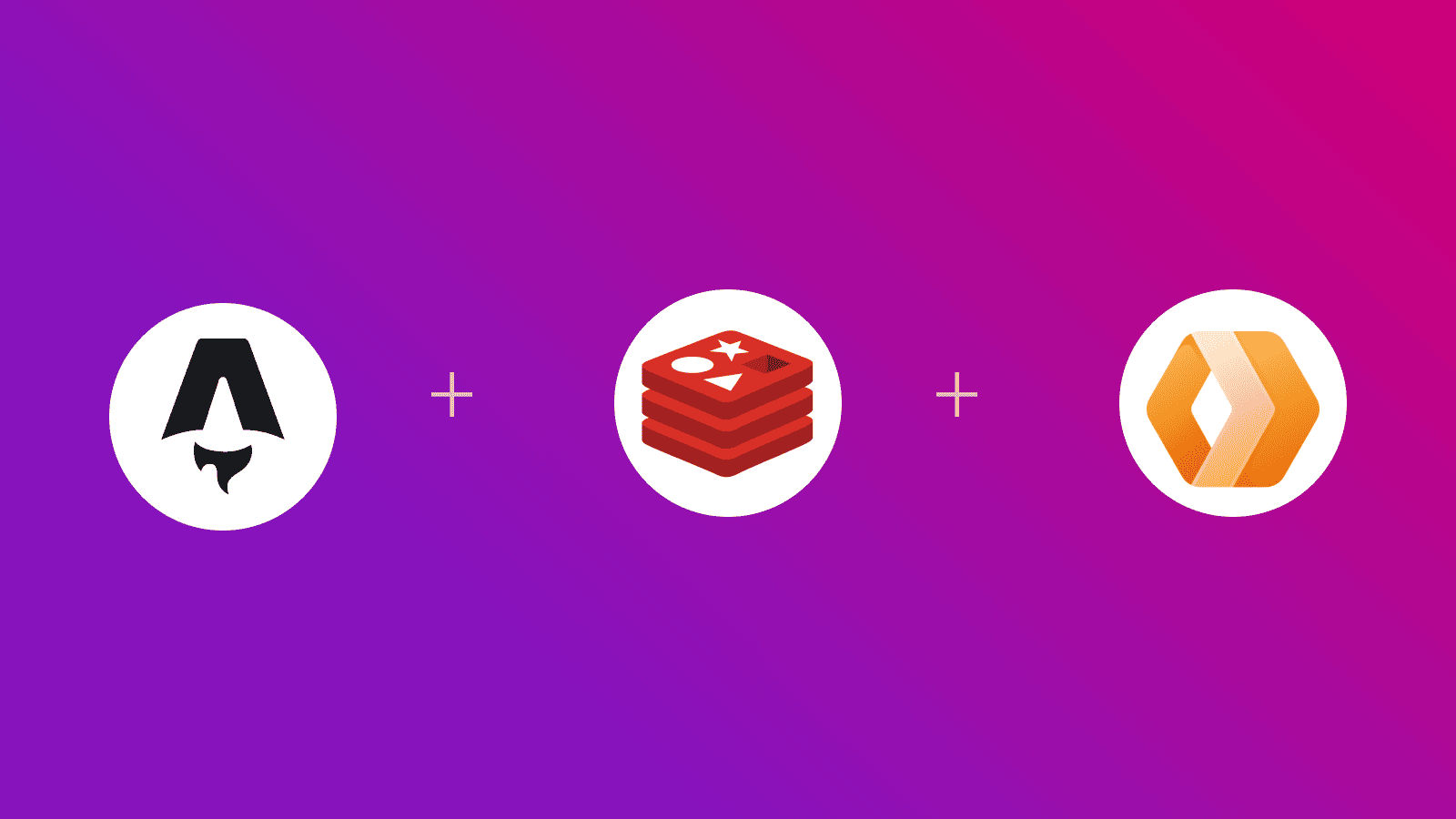
In this guide, you will learn how to interact with any Redis instance from an Astro application running on Cloudflare Workers. You will go through the process of setting up a new Astro project, enabling server-side rendering using Cloudflare adapter, installing the necessary packages, writing code to interact with Redis, and deploy the application to Cloudflare Workers.
Prerequisites
- Node.js 20 or later
- Any Redis database (you can use Upstash for a managed solution)
Table Of Contents
- Create a new Astro application
- Integrate Cloudflare adapter in your Astro project
- Update Type Definitions
- Create a Redis Connection
- Query Redis from Astro application
- Deploy to Cloudflare Workers
Create a new Astro application
Let’s get started by creating a new Astro project. Open your terminal and run the following command:
npm create astro@latest my-app
npm create astro
is the recommended way to scaffold an Astro project quickly.
When prompted, choose:
Use minimal (empty) template
when prompted on how to start the new project.Yes
when prompted to install dependencies.Yes
when prompted to initialize a git repository.
Once that’s done, you can move into the project directory and start the app:
cd my-appnpm run dev
The app should be running on localhost:4321. Next, execute the command below to install the necessary library for building the application:
npm install redis-on-workersnpm install -D tsx
The following libraries are installed:
redis-on-workers
: Connect to your Redis server usingcloudflare:sockets
.tsx
: A runtime for TypeScript and JavaScript that allows you to run TypeScript files directly without needing to compile them first.
Integrate Cloudflare adapter in your Astro project
To deploy your Astro project to Cloudflare Workers, you need to install the corresponding adapter. Execute the command below:
npx astro add cloudflare
When prompted, choose the following:
Y
when prompted whether to install the Cloudflare dependencies.Y
when prompted whether to make changes to Astro configuration file.
You have succesfully enabled server-side rendering in Astro.
To make sure that the output is deployable to Cloudflare Workers, create a wrangler.toml
file in the root of the project with the following code:
name = "redis-astro-workers"main = "dist/_worker.js"compatibility_date = "2025-04-01"compatibility_flags = [ "nodejs_compat" ]
[assets]directory="dist"binding="ASSETS"
[vars]REDIS_URL=""
Post that, make sure that you have both an .env
file and a wrangler.toml
file with the variables defined so that they can be accessed during npm run dev
and when deployed on Cloudflare Workers respectively.
Further, update the astro.config.mjs
file with the following to be able to access these variables in code programmatically:
// ... Existing imports...import { defineConfig, envField } from 'astro/config'
export default defineConfig({ env: { schema: { REDIS_URL: envField.string({ context: 'server', access: 'secret', optional: false }), } } // adapter})
Update Type Definitions
You need to ensure type definitions for the Redis client are correctly applied. For this, create a new file named apply-patch.tsx
in the root of the project with the following code:
import { existsSync, readFileSync, writeFileSync } from 'fs'import { join } from 'path'
const redisWorkersType = join('node_modules', 'redis-on-workers', 'dist', 'index.d.ts')
if (existsSync(redisWorkersType)) { const content = readFileSync(redisWorkersType, 'utf8') if (!content.includes('export declare class RedisInstance')) writeFileSync(redisWorkersType, content.replace('declare class RedisInstance', 'export declare class RedisInstance'))} else console.log(`Warning: Could not find Redis workers type definition at ${redisWorkersType}`)
This code checks if a specific type definition file exists for the Redis client. If it does, the code reads the file and checks for the presence of a specific export. If that export is missing, it modifies the file to add the export.
Now, run the file with the following command:
npx tsx apply-patch.tsx
Create a Redis Connection
In your Astro application, create a file named redis.ts
in the src/storage
directory to establish a connection with Redis. Use the code below:
import { getSecret } from 'astro:env/server'import { createRedis } from 'redis-on-workers'
const connectionString = getSecret('REDIS_URL')if (!connectionString) throw new Error('REDIS_URL is not defined in the environment variables')
export default createRedis(connectionString)
The code above import the getSecret
function from astro:env/server
for accessing environment variables dynamically during the runtime, and defines a redis
variable of type RedisInstance
. It retrieves the Redis connection string from the environment secrets using getSecret, and establishes a Redis connection using createRedis()
if the connection string is available. Finally, it exports the redis instance.
Query Redis from Astro application
Now, you are ready to execute commands on Redis by using the redis
instance. In your Astro page, write the code below to interact with Redis:
---import redis from '../storage/redis'
let productsCount = await redis.sendOnce('GET', 'productsCount')if (!productsCount) productsCount = 0---
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"></head><body> {productsCount}</body></html>
This code imports the redis
instance created earlier and retrieves the value associated with the key productsCount
from Redis using the sendOnce()
method.
Deploy to Cloudflare Workers
To make your application deployable to Cloudflare Workers, create a file named .assetsignore
in the public
directory with the following content:
_routes.json_worker.js
Next, you will need to use the Wrangler CLI to deploy your application to Cloudflare Workers. Run the following command to deploy:
npm run build && npx wrangler@latest deploy
References
Conclusion
In this blog post, you learned how to query a Redis instance from an Astro application running on Cloudflare Workers. You went through the steps of setting up the Redis client, establishing a connection, and executing commands to retrieve data from Redis within the Astro application.
If you would like to explore specific sections in more detail, expand on certain concepts, or cover additional related topics, please let me know, and I’ll be happy to assist!